Laravel Sanctum and Authentication API
motiur15
In modern web application development, user authentication and data security are important requirements. Laravel Sanctum, an extension of Laravel, provides us an easy way to build and manage API authentication system. In this article, we will learn about how to use Laravel Sanctum and apply it to web application development.
Before we start using Laravel Sanctum, we need to install it into our Laravel application. First, run the composer command to install the Sanctum package:
composer require laravel/sanctum
composer require laravel/sanctum
Once the installation is successful, we need to run the Artisan commands to deploy the database to Sanctum:
php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"php artisan migrate
Laravel Sanctum allows us to generate API tokens to authenticate users. This allows users to send API requests containing tokens to confirm their identity and access secure resources.
Here is an example of how to register a user and generate an authentication token in Laravel:
use App\Models\User;use Illuminate\Http\Request;use Illuminate\Support\Facades\Hash;public function register(Request $request){ $request->validate([ 'name' => 'required', 'email' => 'required|email|unique:users', 'password' => 'required|min:6', ]); $user = User::create([ 'name' => $request->name, 'email' => $request->email, 'password' => Hash::make($request->password), ]); $token = $user->createToken('api-token')->plainTextToken; return response()->json(['token' => $token], 201);}
In the above example, we use the createToken method of the user object to create a new authentication token called 'api-token'. We then return that token as JSON so that the user can store it and use it for subsequent API requests.
Once the user has an authentication token, we can use it to authenticate API requests. Laravel Sanctum provides middleware to protect resources that can only be accessed when a valid token is sent with the request.
To protect an API using Sanctum middleware, we need to add 'auth:sanctum' middleware to the respective route or controller. Here is an example of how to protect an API and access user information in the corresponding Route and Controller:
Route::middleware(['auth:sanctum'])->group(function () { // ...});
use App\Http\Controllers\Controller;use Illuminate\Http\Request;class ApiController extends Controller{ public function __construct() { $this->middleware('auth:sanctum'); } public function getUser(Request $request) { $user = $request->user(); return response()->json(['user' => $user], 200); }}
Enjoying the article?
Create a free account to unlock unlimited access to all articles, bookmark your favorites, and join our growing community of readers.
You might also like
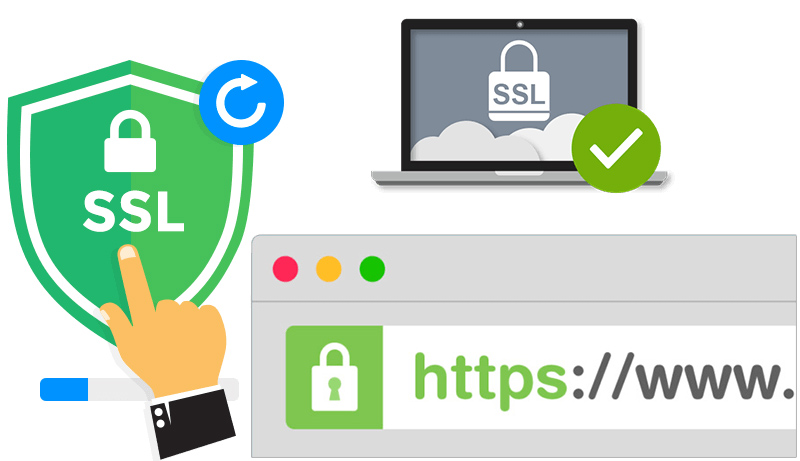
What is a standard SEO website? 30 criteria for evaluating a standard SEO, quality website
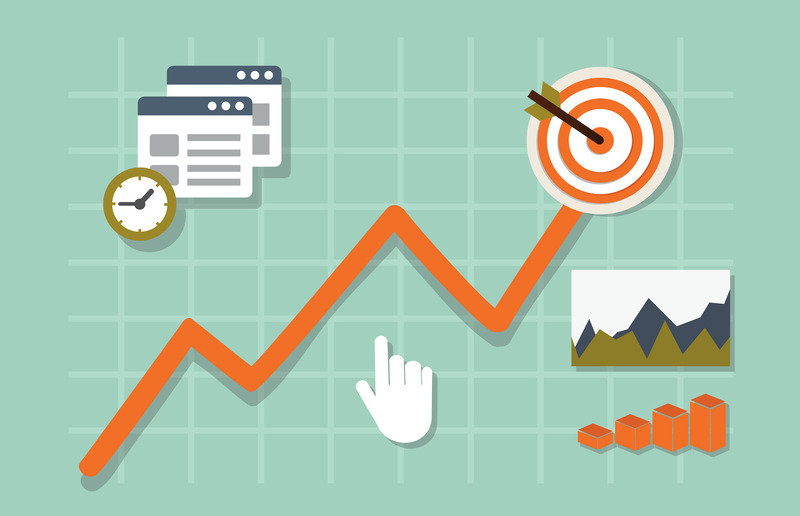
11 ways to increase website traffic safely and effectively
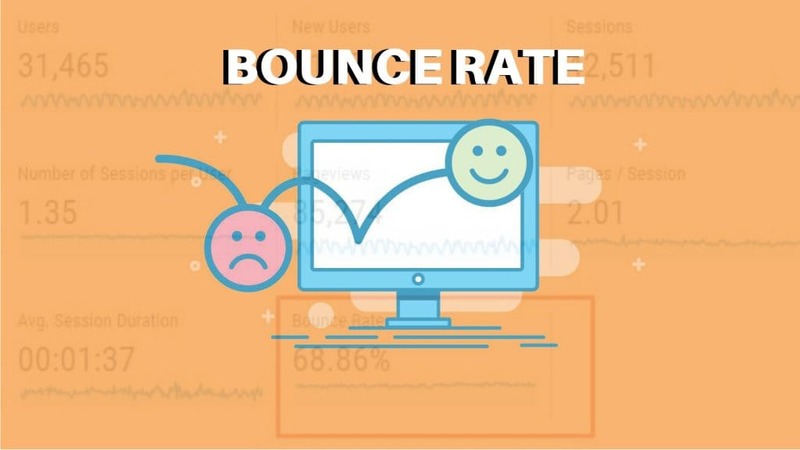
What is bounce rate? How much is good and how to optimize bounce rate effectively
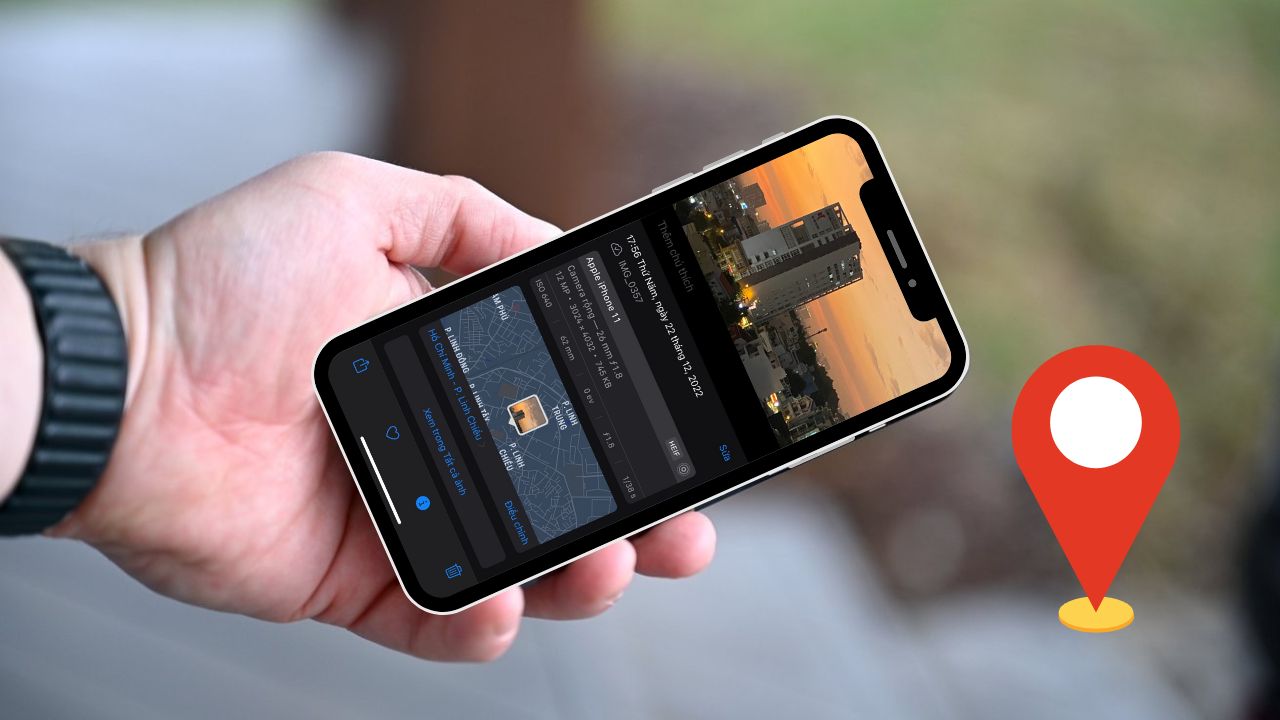
What is photo geotagging? How to optimize and geotag photos effectively and professionally
motiur15
Want to Share Your Knowledge?
Join our community of writers and help others learn from your experience. Start writing today.
Get Started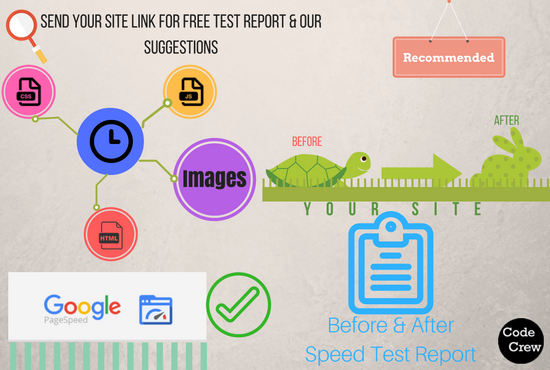
Optimize WordPress Speed with PageSpeed Insights
Boost Your WordPress Site Speed with Google PageSpeed – First Customer Gets 75%...
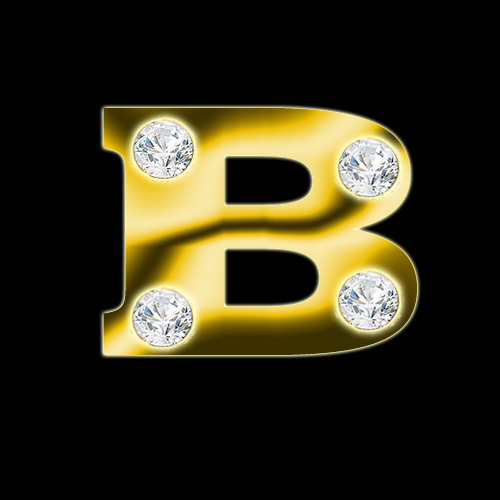
Stunning 24K Gold Textured Alphabets Design
ELEVATE YOUR PROJECTS WITH UNPARALLELED 24K GOLD TEXTURIZED ALPHABETS AND DIAMON...
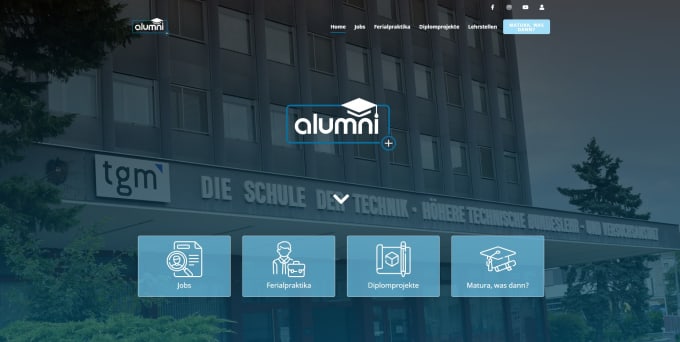
Build Stunning Premium WordPress Websites with Elementor Pro
Craft a Stunning and SEO-Optimized Premium WordPress Site with Elementor ProAr...
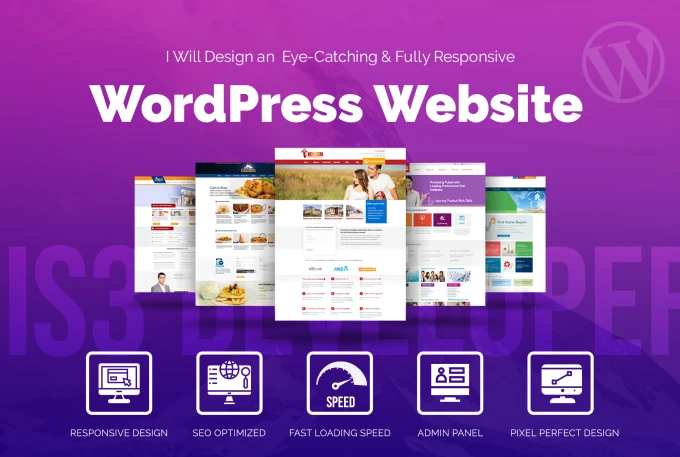
Create Engaging WP Membership/Learning Site Easily
Craft a Dynamic WordPress Membership or E-Learning WebsiteLooking to build a r...

Professional On-Page SEO & Technical Optimization for Top Google Rankings
Are you searching for comprehensive On-page SEO and technical optimization servi...
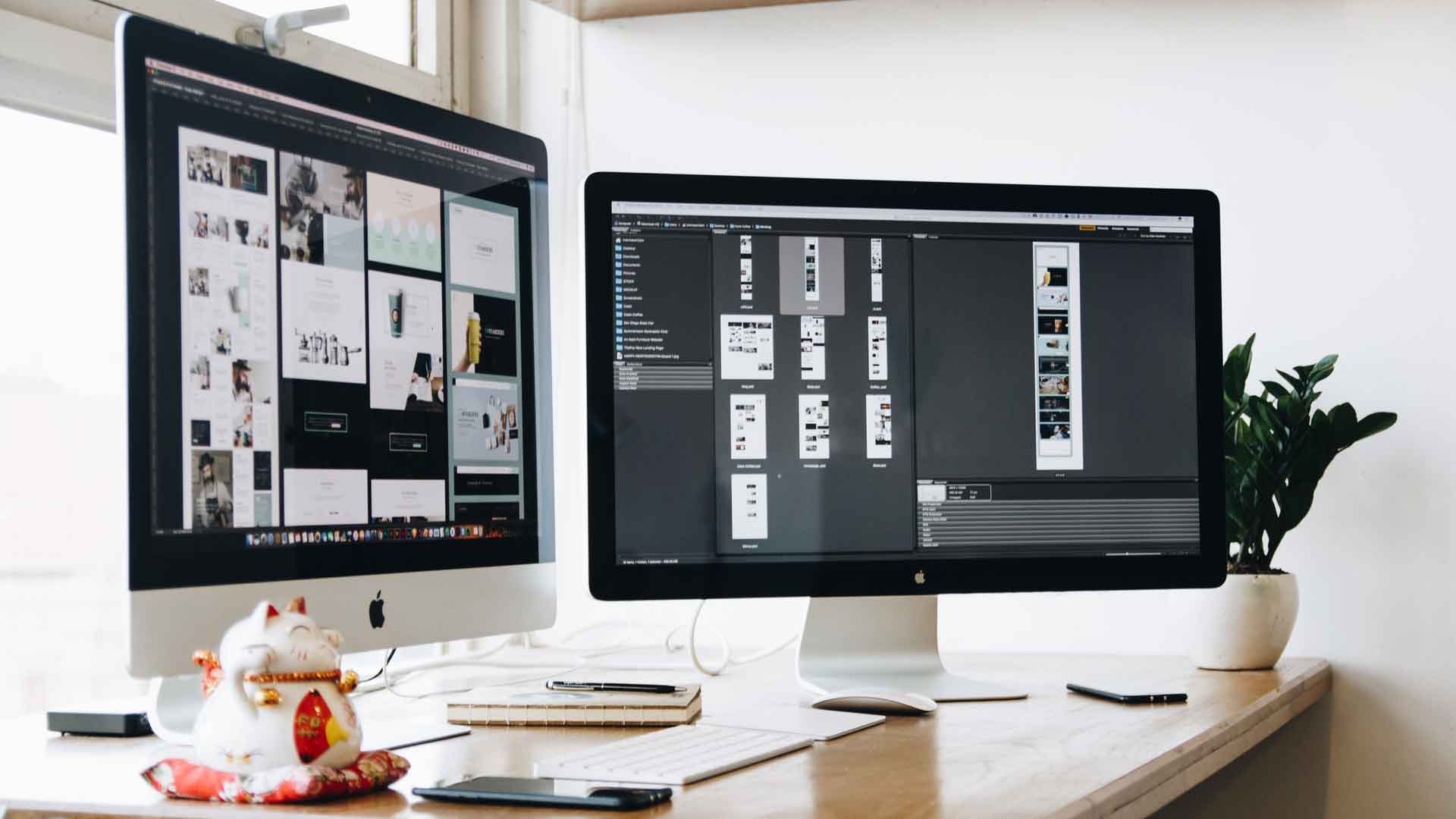
3-Page WP Site + 3 Pro Plugins Free!
Craft a Stunning 3-Page WordPress Site with Essential 3 Pro Plugins IncludedDes...
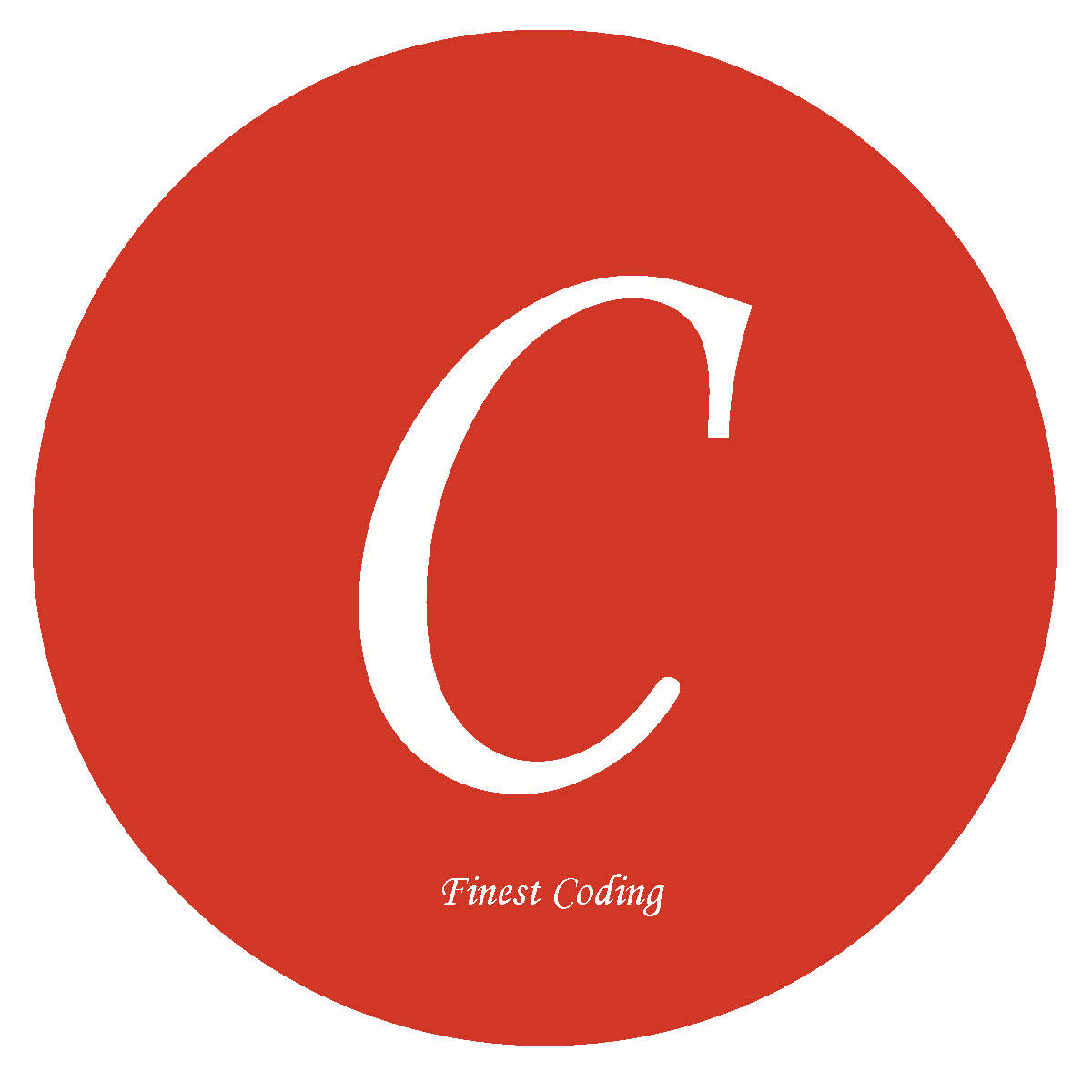
Professional WordPress Maintenance & Development
WordPress Expert ServicesServices Include:- Comprehensive Backups and Manual U...
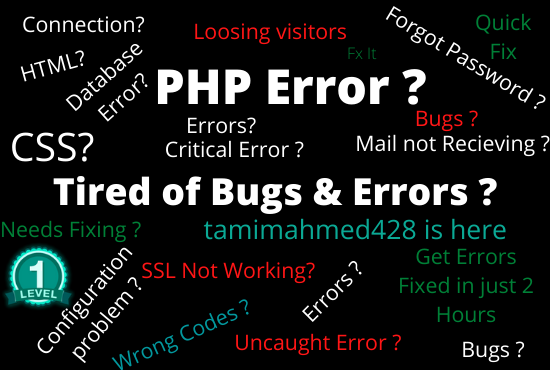
WordPress Fixes Within 2 Hours
I specialize in resolving all types of WordPress issues within 2 hours!Hello,...
Latest Articles
Optimize the speed of your Laravel application
3 weeks ago • rakiburdma
SWOOLE Installation and Configuration
3 weeks ago • shiktocf
Open Swoole – An extremely fast PHP asynchronous processing framework
3 weeks ago • shiktocf
What is Heading? Tips to SEO-optimize effective Heading for beginners 2025
4 weeks ago • iBial Team
Onpage SEO 2025? 26 Onpage SEO optimization criteria from basic to advanced
4 weeks ago • iBial Team