Optimize the speed of your Laravel application
rakiburdma
We all agree that no one wants to use a slow application. What we want is “fast”.
According to a Google study, 53% of mobile users leave a website that takes more than 3 seconds to load. So performance is critical.
Every second your website takes to load is a loss of users. Fortunately, with Laravel, this is not a difficult problem to solve, so let's learn how to improve the performance of websites built with Laravel.
Using Laravel's built-in features
Laravel provides a number of built-in features to improve performance for any website built on it.
Using cache is a very popular performance improvement method and Laravel also supports it, typically route caching. You can see that every time you access the website, the system behind the scenes will perform a series of actions to return the user's display requests. So each load will be performed from the beginning and take that much time to return the information the user needs.
So what you need is to store the returned result in a place called cache. Specifically here we will save the routing processing information of Laravel by using the following Artisan command:
php artisan route:cache
This command can increase your website performance up to 5x. It has a pretty significant impact on your website.
Besides route:cache, Laravel also provides other caches such as:
- View cache
- Config cache
- Event cache
Select the appropriate Drivers
Choosing the right cache, queue, and session drivers also increases your web application performance.
In production, we recommend using cache drivers like Redis, Memcached, or DynamoDB.
For queues you can use Redis, SQS, or Beanstalkd drivers which will give significant performance gains.
For sessions you should use Database, Redis, Memcached, or DynamoDB drivers. You should not use cookie drivers in production due to security concerns.
Remove Unused Service
You should not load unnecessary services through config, but note to only load necessary configs and disable unused services in the config file.
Classmap Optimization
You can increase performance by merging included files to combine them into one file so that when calling include file will only call and use one file without reading many different files which wastes system time. In Laravel you use the following command to do this:
php artisan optimize --force
Note: Only use with version below 5.5, new version will automatically integrate without command.
Use libraries wisely
Using too many libraries will slow down your website because it has to handle many information streams of different libraries. So if you don’t use any library, remove it to improve performance. There are two places you need to find and remove unused libraries: config/app.php and composer.json.
Query result cache
You should cache frequently used queries that return the same results to reduce database queries and increase performance:
$posts = Cache::remember('index.posts', 30, function() { return Post::with('comments', 'tags', 'author', 'seo')->whereHidden(0)->get();});
Use “Eager Loading”
You already know that n+1 expression increases the number of queries and reduces performance. When used in combination with queries, it will reduce your performance significantly if the number of iterations is large. To solve this, use Eager Loading:
// slow performance (bad)$post = App\Articles::all();foreach ($ as $post) { echo $post->source->uri;}// eager loading (good)$post = App\Articles::with('source')->get();foreach ($post as $post) {echo $post->source->uri;}
Use CDN
Using CDN for your website also significantly increases performance for your web users by automatically caching your static, unchanging content such as images, js, css.
CDN allows quick access to website data like cache without having to retrieve data on the production server. It is also suitable for websites with users in many different access locations.
Assets minification
Use Laravel mix to help optimize css, js, image files, ... to reduce the size of these files, thereby increasing the speed of reading files, making your website faster. To use it, you need to install nodejs and npm. Then use only the following command to succeed:
npm run production
Latest version
Using the latest version of PHP or Laravel also helps you improve the performance of your web application, because the latest version will have many major changes in features as well as enhance its performance.
Conclude
So I have shown you the basic ways to significantly improve the performance of your Laravel website. There are also many other ways to speed up your Laravel website. Please find out more and share it with everyone.
You might also like
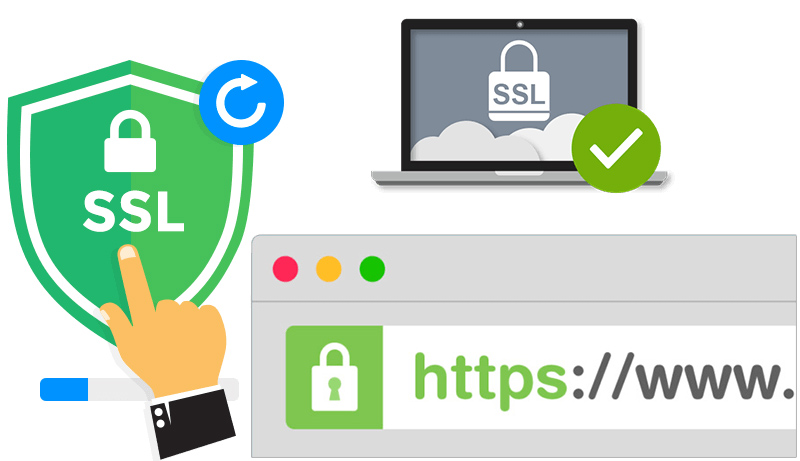
What is a standard SEO website? 30 criteria for evaluating a standard SEO, quality website
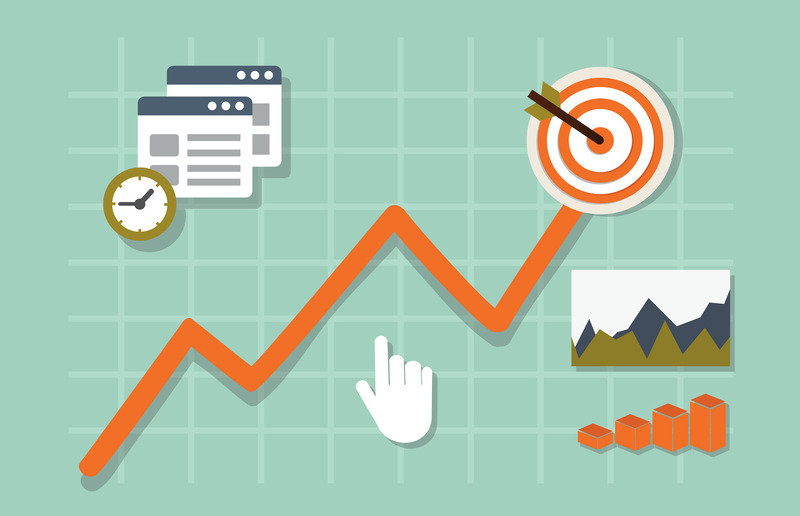
11 ways to increase website traffic safely and effectively
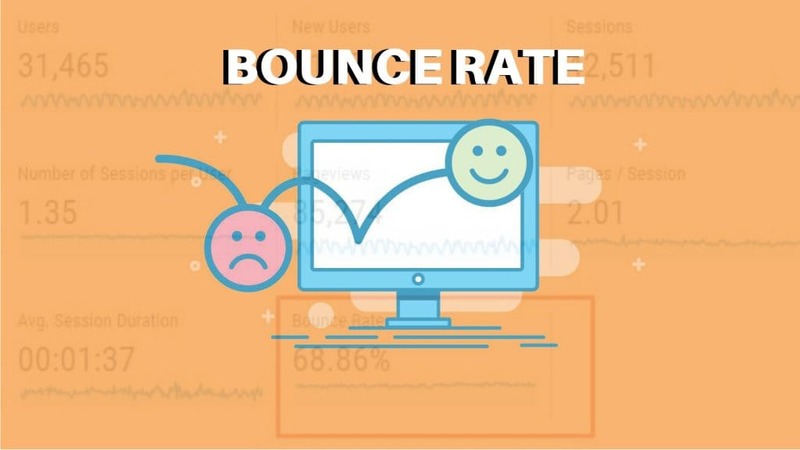
What is bounce rate? How much is good and how to optimize bounce rate effectively
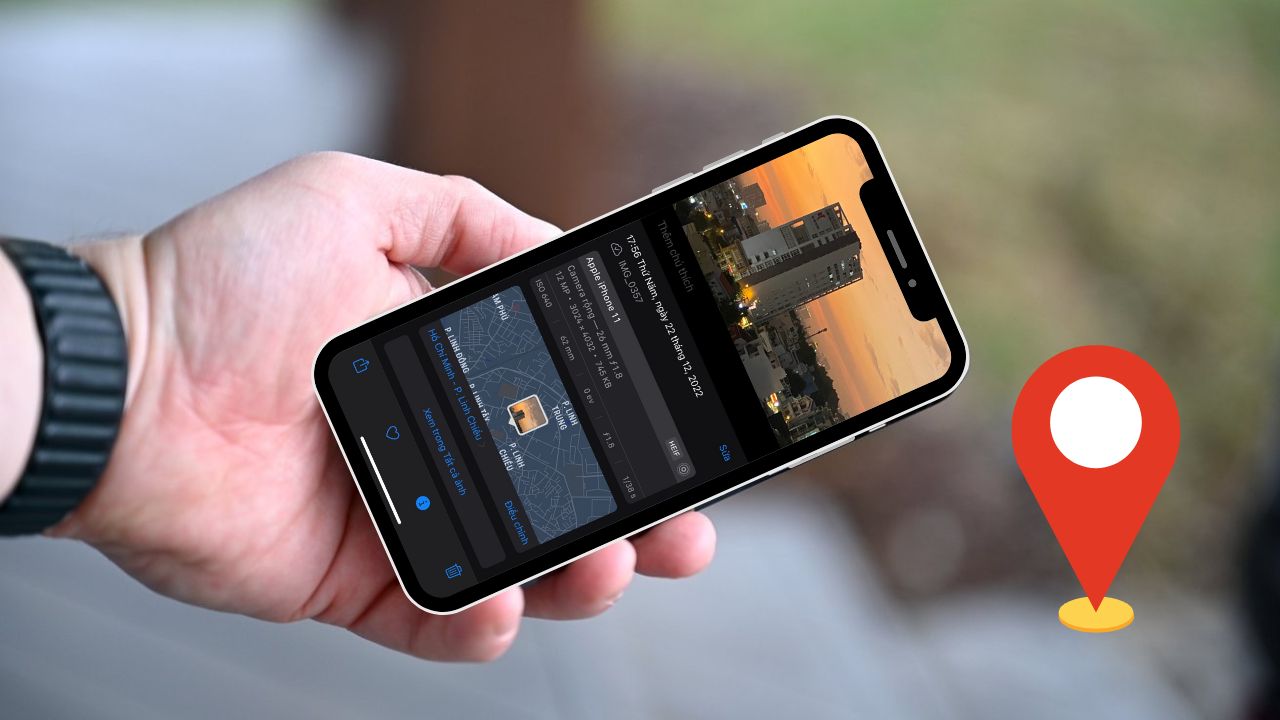
What is photo geotagging? How to optimize and geotag photos effectively and professionally
WordPress Site Speed Optimized Within 24 Hours
Optimize Your WordPress Website for Speed Within 24 HoursNeed a speedy and optimized WordPress web...
Top-Grade Hosting + .com Domain
Embrace the Global Stage with Premium Web Hosting and .com DomainIn an increasingly interconnected...
Expert WordPress Website Design & SEO Optimization
EXPERT WP DEVELOPER: Crafting User-Friendly & SEO-Powered WebsitesMeet Marvin, your go-to WordPres...
Build Stunning WordPress Website with Divi/Elementor
Transform Your Vision into a Stunning WordPress WebsiteWith over five years of experience in WordP...
rakiburdma
Want to Share Your Knowledge?
Join our community of writers and help others learn from your experience. Start writing today.
Get Started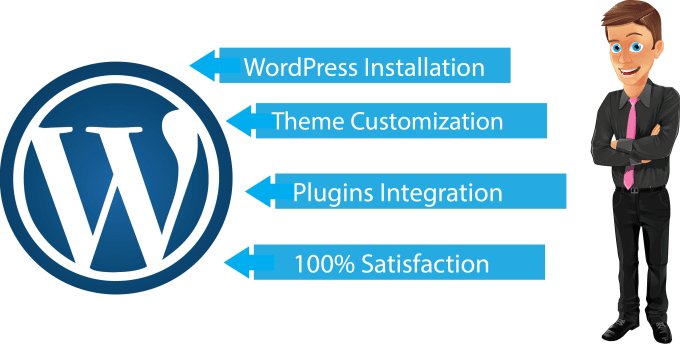
Professional WordPress Customization Services
I specialize in a wide range of WordPress customization and maintenance tasks to...
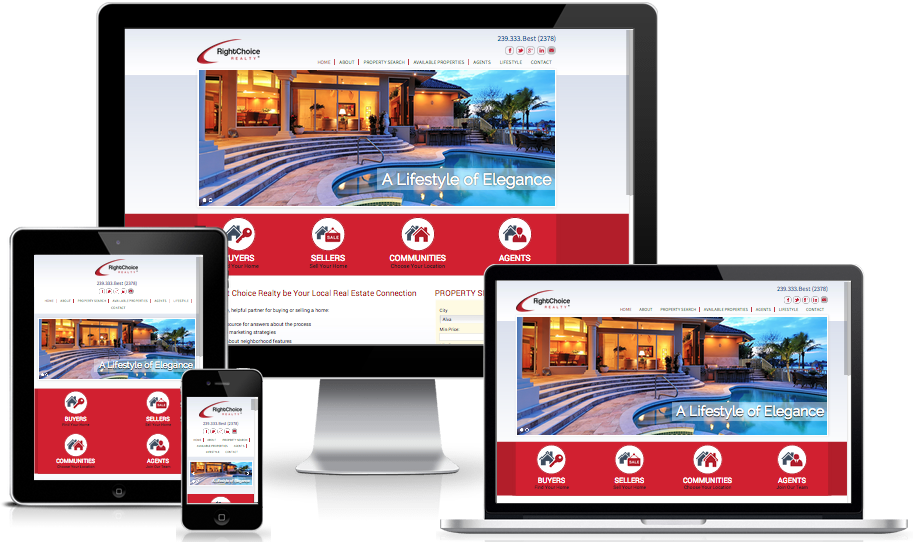
Create Your Dream Blog/E-Commerce Site
Craft Your Ideal Blog or E-Commerce Site with UsAbout Us:With over 4 years of...
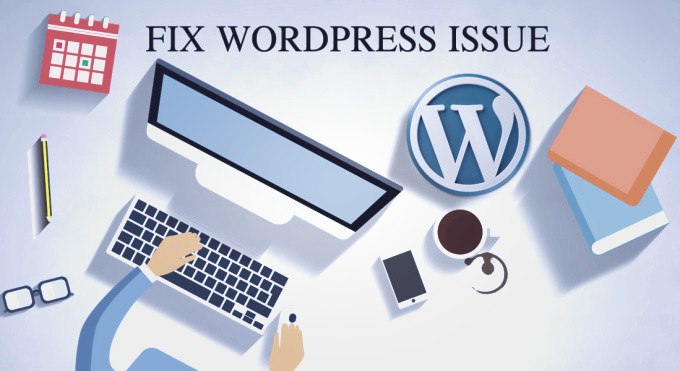
Fix WordPress Bugs Rapidly
Solve WordPress Bugs Within 24 HoursWordPress might be user-friendly, but it's...
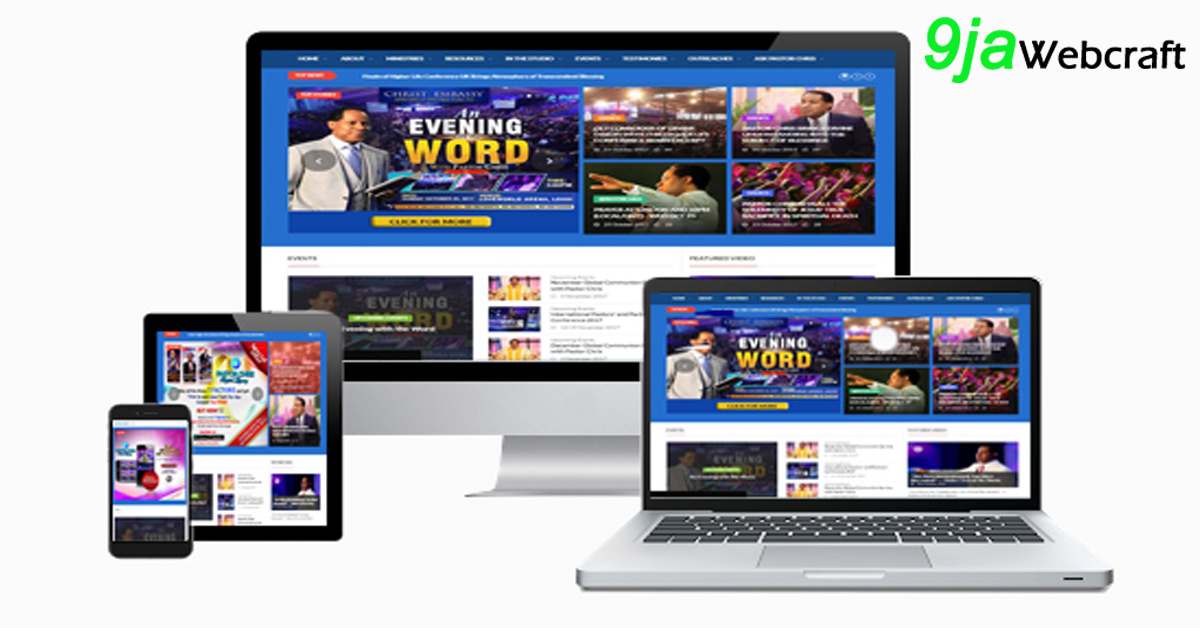
Expert WordPress Website Design & SEO Optimization
EXPERT WP DEVELOPER: Crafting User-Friendly & SEO-Powered WebsitesMeet Marvin,...

Fix WordPress Errors & Issues Swiftly
Elevate Your WordPress Experience with Expert Troubleshooting and Custom Solutio...
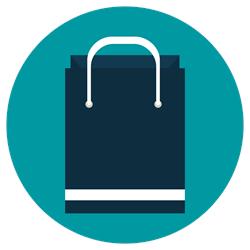
Build API Integration & Amazon Affiliate Store
Expert Services: API Integration & Amazon Affiliate Store CreationHello! I'm N...
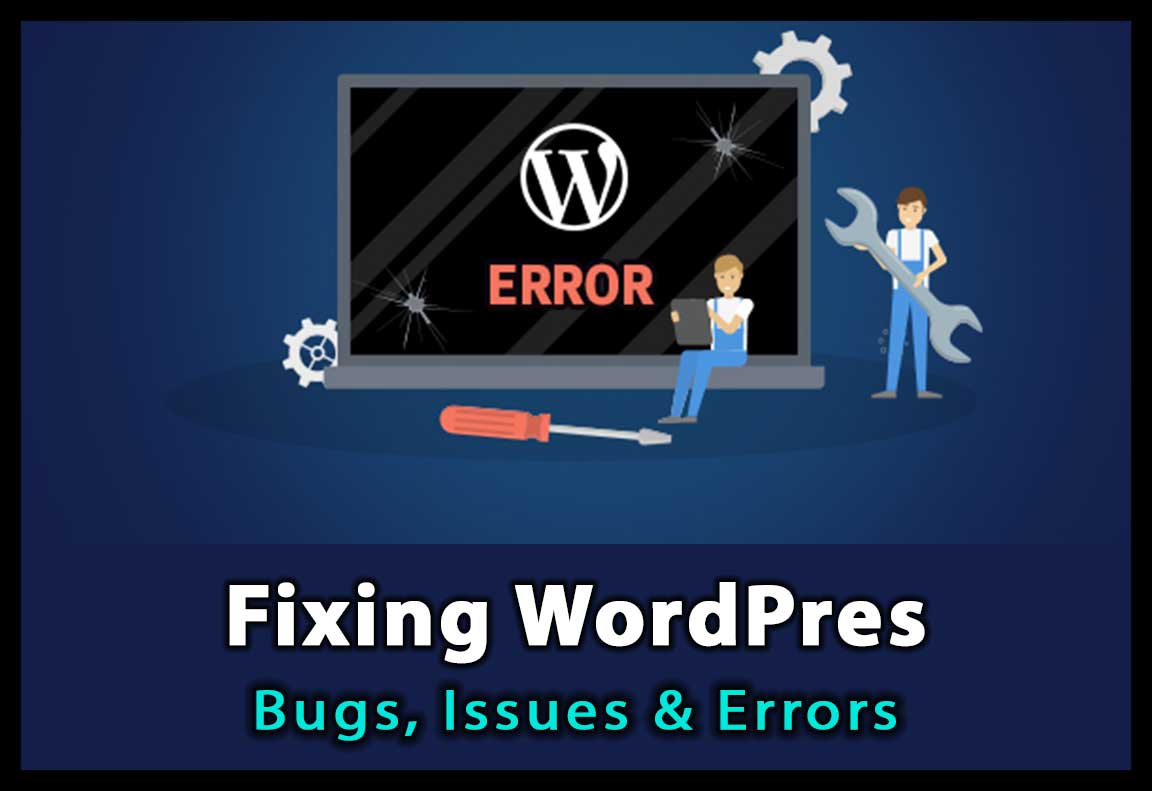
Fix All WordPress Bugs, Issues, Errors
I specialize in resolving any WordPress bugs, issues, or errors you might encoun...
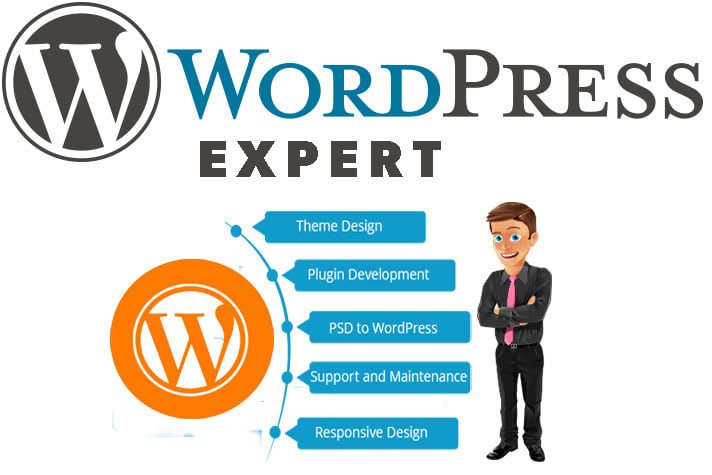
Custom WordPress Site From Your Design
Create Your Dream WordPress Website Today!With my Master’s degree in Computer S...
Latest Articles
SWOOLE Installation and Configuration
3 weeks ago • shiktocf
Open Swoole – An extremely fast PHP asynchronous processing framework
3 weeks ago • shiktocf
What is Heading? Tips to SEO-optimize effective Heading for beginners 2025
4 weeks ago • iBial Team
Onpage SEO 2025? 26 Onpage SEO optimization criteria from basic to advanced
4 weeks ago • iBial Team
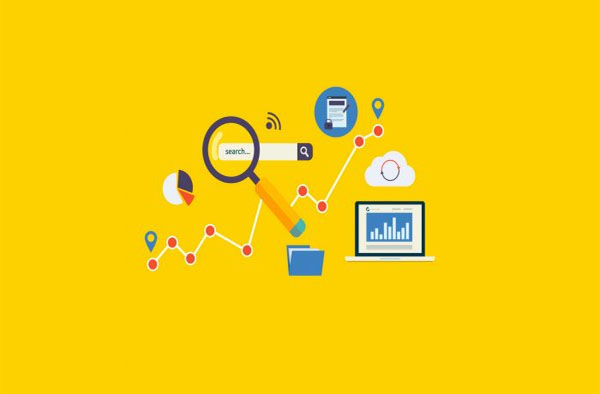
What is organic traffic? Reasons and ways to invest in organic traffic effectively
4 weeks ago • pial